Table of Contents
What is Floyd Algorithm?
Floyd algorithm finds the shortest paths in a weighted graph with positive or negative edge weights. It can also detect negative cycles.
The algorithm works by starting at a given vertex and then using a relaxation technique to gradually find the shortest path between that vertex and all other vertices in the graph. The relaxation step works by considering each edge in the graph and updating the cost associated with each vertex if a shorter path is found.
When all edges have been considered, the shortest paths between the given vertex and all other vertices will have been found. The algorithm can then be repeated for all other vertices in the graph to find the shortest paths between any two vertices.
It is important to note that Floyd’s algorithm can only be used on graphs with positive or negative edge weights. If any of the edge weights are zero, then the algorithm will not work as expected.
How Does The Floyd Algorithm Work?
The algorithm works by iteratively computing the shortest path from each node to every other node using the “relaxation” technique. The algorithm is capable of handling both directed and undirected graphs, as well as mixed graphs containing both positive and negative weights.
In each iteration, the algorithm relaxes all edges that could lead to a shorter path. This process is repeated until no further relaxation is possible and the shortest paths between all pairs of nodes have been determined.
The Floyd algorithm is widely used in network routing protocols and for solving the All-Pairs Shortest Paths (APSP) problem. It is also used in various applications such as finding the transitive closure of a relation, constructing shortest-path spanning trees, and computing the distance matrix of a graph.
The algorithm works by iteratively relaxing the shortest path estimates between all pairs of vertices in the graph. Specifically, it maintains a distance matrix D, where D [i] [j] is the shortest distance from vertex i to vertex j.
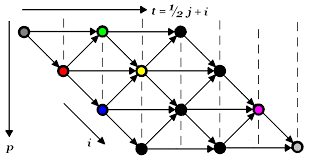
The algorithm repeatedly updates this matrix by considering all pairs of vertices (i, j) and checking if there is a path from i to j that is shorter than the current shortest path estimate. If such a path exists, the algorithm updates the distance estimate for the pair (i, j) accordingly.
The algorithm has a time complexity of O(n^3), where n is the number of vertices in the graph. This makes it suitable for use on dense graphs, but not on very large charts or graphs with a high number of edges.
Here is some pseudocode for the Floyd algorithm:
function floyd(graph):
n = len(graph)
D = copy(graph) # Initialize distance matrix with the graph
for k in range(n):
for i in range(n):
for j in range(n):
D[i][j] = min(D[i][j], D[i][k] + D[k][j]) # Relax the shortest path estimate
return D
This pseudocode assumes that the input graph is represented as an adjacency matrix, where graph [i] [j] is the weight of the edge from vertex i to vertex j, or infinity if there is no such edge. The function returns the distance matrix D, where D [i] [j] is the shortest distance from vertex i to vertex j.
Certainly! Here is a simple example of the Floyd algorithm on a small graph with three vertices:
A —– B
| |
5| | 2
| |
C —– D
3
To find the shortest paths between all pairs of vertices in this graph, we can use the following steps:
Initialize the distance matrix D with the graph itself. We can represent the graph as an adjacency matrix as follows:
D = [[0, 5, INF],
[INF, 0, 2],
[INF, INF, 0]]
Here, INF represents infinity, and 0 represents the fact that there is no distance between a vertex and itself.
Iterate over all pairs of vertices (i, j) and check if there is a path from i to j that is shorter than the current shortest path estimate. Specifically, we check if D[i][j] > D[i][k] + D[k][j] for any intermediate vertex k. If such a path exists, we update the distance estimate for the pair (i, j) accordingly.
For example, in the first iteration, we consider the pair (A, C). We check if there is a shorter path from A to C via an intermediate vertex B. Since D[A][C] = INF and D[A][B] + D[B][C] = 5 + 2 = 7, we update D[A][C] to be equal to D[A][B] + D[B][C] = 7.
We continue this process until we have considered all pairs of vertices. After all iterations, the distance matrix D will contain the shortest distance between all pairs of vertices in the graph:
D = [[0, 5, 7],
[INF, 0, 2],
[INF, INF, 0]]
I hope this example helps to clarify how the Floyd algorithm works.
The Use And Applications Of The Floyd Algorithm
The Floyd algorithm is a general-purpose algorithm for finding the shortest paths in a weighted graph. It can be used in a variety of applications where it is important to find the shortest path between two vertices in a graph, such as:
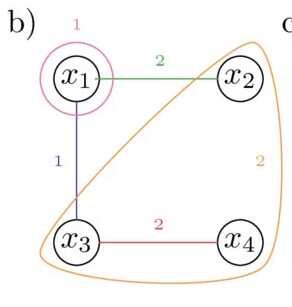
- Network routing: The Floyd algorithm can be used to find the shortest path between two nodes in a communication network, such as a computer network or a transportation network.
- Map routing: The Floyd algorithm can be used to find the shortest path between two points on a map, taking into account factors such as road distance and traffic.
- Project scheduling: The Floyd algorithm can be used to find the shortest path through a project network, taking into account the dependencies between tasks and the time required to complete each task.
- Data compression: The Floyd algorithm can be used to find the shortest path through a tree data structure, which can be used to compress data.
- Social network analysis: The Floyd algorithm can be used to find the shortest path between two individuals in a social network, taking into account the relationships between individuals.
These are just a few examples of the many possible applications of the Floyd algorithm.
The Floyd algorithm is a useful tool for finding the shortest paths in a weighted graph with positive or negative edge weights (but with no negative cycles). It works by iteratively relaxing the shortest path estimates between all pairs of vertices in the graph and has a time complexity of O(n^3), where n is the number of vertices in the graph.
This makes it suitable for use on dense graphs but not on very large charts or graphs with a high number of edges. The Floyd algorithm has a wide range of applications, including network routing, map routing, project scheduling, data compression, and social network analysis. I hope this summary helps to clarify the main points about the Floyd algorithm.
FAQ
What is the Floyd algorithm?
The Floyd algorithm, also known as the Floyd-Warshall algorithm, is a graph algorithm used to find the shortest paths between all pairs of vertices in a weighted graph. It was developed by Robert Floyd and Stephen Warshall in the early 1960s.
How does the Floyd algorithm work?
The Floyd algorithm uses dynamic programming to calculate the shortest paths between all pairs of vertices. It builds a matrix of distances between vertices, updating the distances iteratively until it finds the shortest paths.
What is the time complexity of the Floyd algorithm?
The time complexity of the Floyd algorithm is O(V^3), where V is the number of vertices in the graph. It needs to consider all pairs of vertices and perform updates for each pair, resulting in a cubic time complexity.
Can the Floyd algorithm handle negative-weight edges?
Yes, the Floyd algorithm can handle negative-weight edges. However, if the graph contains negative-weight cycles, the algorithm may not produce correct results.
What is the significance of the Floyd algorithm?
The Floyd algorithm is significant because it solves the all-pairs shortest path problem efficiently. It can be used to find the shortest paths in a graph, which has applications in various fields such as transportation, network routing, and data analysis.
Does the Floyd algorithm work for directed and undirected graphs?
Yes, the Floyd algorithm works for both directed and undirected graphs. It considers the weights of edges in the graph to find the shortest paths between all pairs of vertices, regardless of the direction of the edges.
Can the Floyd algorithm handle graphs with cycles?
Yes, the Floyd algorithm can handle graphs with cycles. It considers all possible paths between pairs of vertices, including those that may involve cycles, and updates the distances accordingly.
Are there any alternatives to the Floyd algorithm?
Yes, there are alternative algorithms to find the shortest paths in a graph, such as Dijkstra’s algorithm and the Bellman-Ford algorithm. These algorithms are more efficient when you need to find the shortest path from a single source vertex to all other vertices.
Does the Floyd algorithm guarantee the optimal shortest paths?
Yes, the Floyd algorithm guarantees the optimal shortest paths between all pairs of vertices. It considers all possible paths and updates the distances until it finds the shortest paths.
Can the Floyd algorithm be used for graphs with a large number of vertices?
The Floyd algorithm has a cubic time complexity, which makes it less efficient for graphs with a large number of vertices. For such cases, alternative algorithms like Dijkstra’s algorithm or A* algorithm may be more suitable, as they have better time complexities for finding the shortest paths from a single source vertex.
Also, see Computer Engineering