Table of Contents
Encapsulation is a fundamental concept in object-oriented programming, and it plays a critical role in C++. Encapsulation refers to the practice of binding data and functions together and restricting access to them from outside the class. In simpler terms, it means that the internal workings of an object are hidden from the outside world, and only the necessary information is exposed.
C++ provides several mechanisms to implement encapsulation, and understanding these mechanisms is essential for writing robust and secure code. In this article, we will explore encapsulation in C++ and its importance in object-oriented programming.
Encapsulation in C++: The Basics
The primary mechanism for implementing encapsulation in C++ is the use of access specifiers. C++ provides three access specifiers: private, protected, and public. These access specifiers define the level of access that the data members and member functions of a class have.
Private Access Specifier
The private access specifier restricts access to the data members and member functions to only the class itself. This means that these members cannot be accessed from outside the class, including other classes and functions. Private members can only be accessed by the member functions of the class.
Protected Access Specifier
The protected access specifier is similar to the private access specifier in that it restricts access to the data members and member functions to only the class and its derived classes. This means that these members cannot be accessed from outside the class hierarchy.
Public Access Specifier
The public access specifier provides unrestricted access to the data members and member functions of a class. This means that these members can be accessed from anywhere, including outside the class hierarchy.
Benefits of Encapsulation
Encapsulation offers several benefits, including data hiding, modularity, and reusability. By hiding the internal workings of an object, encapsulation ensures that the data is protected from unauthorized access and modification. This promotes data integrity and security, which is crucial in many applications.
Encapsulation also promotes modularity by separating the implementation details of an object from its interface. This allows for the development of complex systems with many interacting components without exposing the implementation details to the outside world.
Finally, encapsulation promotes reusability by providing a clean and well-defined interface to the outside world. This allows for the creation of reusable components that can be used in many different contexts without requiring any knowledge of their implementation details.
Encapsulation is a critical concept in object-oriented programming, and it plays a crucial role in C++. The use of access specifiers allows for the implementation of data hiding, modularity, and reusability, which are essential for writing robust and secure code. By understanding the principles of encapsulation, developers can write better software that is more reliable, more secure, and more maintainable.
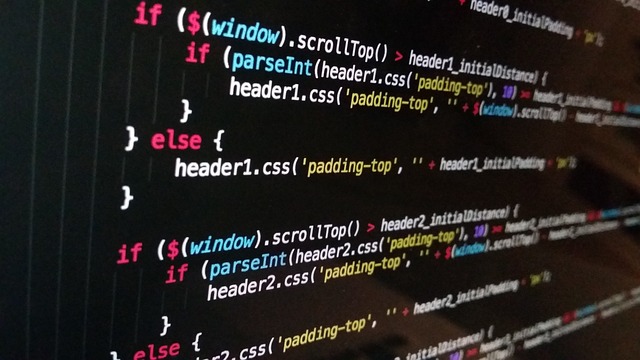
What is encapsulation in C++ with real-life examples?
Encapsulation in C++ is a fundamental object-oriented programming concept that refers to the practice of bundling data and the methods that operate on that data within a single entity, known as a class. The primary goal of encapsulation is to hide the internal details of a class from the outside world, providing a well-defined interface for interacting with the class’s behavior.
Here are some real-life examples that can help you understand encapsulation in C++:
Bank Account: A bank account is a classic example of encapsulation. The account holder is only allowed to access their own account, and they can perform specific operations such as deposit and withdrawal, while the bank’s internal mechanisms remain hidden from the user.
Car: A car’s internal workings, such as the engine and transmission, are encapsulated within the car’s body. A driver interacts with the car’s interface, such as the accelerator, brake, and steering wheel, without needing to know the details of how the car’s components work together.
Smartphone: A smartphone has various functionalities such as making phone calls, sending text messages, and accessing the internet. The user interacts with the smartphone’s interface, such as the touchscreen and buttons, while the internal details of how the phone works remain hidden from the user.
In each of these examples, encapsulation provides a clear separation of concerns between the interface and the implementation of a class, promoting modularity, and allowing changes to be made to the internal workings of a class without affecting the class’s interface.
What is encapsulation in OOP?
Encapsulation is one of the fundamental principles of object-oriented programming (OOP) that describes the concept of bundling data and methods into a single unit and restricting access to the inner workings of that unit from outside the object.
In other words, encapsulation means wrapping the implementation details of an object within its interface and providing a well-defined interface for the outside world to interact with the object. This allows objects to maintain their integrity and protect their state from being unintentionally modified by external entities.
In practice, encapsulation is achieved by defining class members (variables and methods) with different access levels (public, private, protected) that specify which parts of the class are visible and accessible from outside. For example, public members can be accessed from any part of the program, private members can only be accessed from within the class, and protected members can be accessed from within the class or its subclasses.
By using encapsulation, OOP promotes the principles of abstraction, modularity, and information hiding, which can make code more robust, maintainable, and easier to understand and modify.
What is the purpose of encapsulation in C++?
Encapsulation is a fundamental concept in object-oriented programming and refers to the practice of bundling related data and methods within a single unit or module to restrict access to the internal workings of an object. In C++, encapsulation is achieved through the use of access modifiers, such as public, private, and protected, which determine how the members of a class can be accessed by code outside of the class.
The purpose of encapsulation in C++ is to provide a mechanism for hiding the implementation details of an object from the rest of the program. This allows developers to change the implementation of an object without affecting the rest of the program. By making the data members of a class private and providing public member functions to access and manipulate them, developers can ensure that the object’s internal state is not corrupted by external code.
Encapsulation also promotes code reusability and modularity by allowing developers to create classes with well-defined interfaces that can be easily reused in other parts of a program or in other programs altogether. This also makes it easier to debug and maintain code since the internal state of an object is hidden and cannot be accessed or modified directly by external code.
Overall, encapsulation is a crucial concept in C++ and other object-oriented programming languages as it provides a mechanism for creating robust, modular, and maintainable code.
What are the different types of encapsulation?
In computer science, encapsulation is a technique of hiding implementation details of an object or module from the outside world and providing access to it through a well-defined interface. There are two main types of encapsulation:
Data Encapsulation:
Data encapsulation is the process of hiding the internal data and implementation details of an object from the outside world and providing a public interface to access and modify the data. In other words, it refers to the practice of bundling data and the methods that operate on that data within a single unit.
For example, in object-oriented programming, a class can be used to encapsulate data and related methods. The data members of the class are declared private, which means they cannot be accessed directly from outside the class. Instead, public methods are defined to access and modify the private data members.
Abstraction Encapsulation:
Abstraction encapsulation is a more general concept that encompasses data encapsulation, as well as other techniques of hiding implementation details from the outside world. Abstraction refers to the process of identifying and separating the essential features of an object or system from the non-essential details.
For example, in software engineering, abstraction encapsulation can be used to separate the interface of a module from its implementation details. This allows the module to be changed or replaced without affecting the rest of the system, as long as the interface remains unchanged.
Data encapsulation is a specific type of encapsulation that focuses on hiding the implementation details of an object’s data, while abstraction encapsulation is a more general concept that encompasses a broader range of techniques for hiding implementation details.
Also, read Prime number program in c++